일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- 스프링
- method refetence
- 스프링부트 도커
- 비기능적 요구사항
- open-session-in-view
- 생성자주입
- jpa no session
- kotlin 리팩터링
- 소프트웨어의 품격
- jpa lazy
- 그래프큐엘
- 도커 이미지 빌드
- 스프링시큐리티
- fetch join
- 자바 필터
- 스프링 포매터
- Spring
- ioc컨테이너
- java predicate
- spring formatter
- 동적파라미터
- 토비의 스프링
- 정적팩토리메서드
- Atomicity
- 스프링 시큐리티 설정
- 수정자주입
- 스프링di
- 기능적 요구사항
- IOC
- kotlin ::
Archives
- Today
- Total
공부기록
[JUnit5] 기본 테스트 어노테이션(@Test, @BeforeAll, @BeforeEach, @AfterAll, @AfterEach, @Disabled) 본문
Test
[JUnit5] 기본 테스트 어노테이션(@Test, @BeforeAll, @BeforeEach, @AfterAll, @AfterEach, @Disabled)
gracelove91 2020. 8. 13. 04:18반응형
본 포스팅은 백기선님의 "더 자바, 애플리케이션을 테스트하는 다양한 방법" 을 보고 정리한 글 입니다.
관심 있으신 분들은 https://www.inflearn.com/course/the-java-application-test 를 살펴보세요
개요
Junit5의 기본 애너테이션이라고 할 수 있는 @Test
, @BeforeAll
, @BeforeEach
, @AfterAll
, @AfterEach
, @Disabled
를 알아보자.
@Test
본 어노테이션을 붙이면 Test 메서드로 인식하고 테스트 한다.
JUnit5 기준으로 접근제한자가 Default 여도 된다. (JUnit4 까지는 public이어야 했었다.)
@Test
void create1() {
Study study = new Study();
assertNotNull(study);
System.out.println("create1()");
}
@Test
void create2() {
System.out.println("create2()");
}
@BeforeAll
본 어노테이션을 붙인 메서드는 해당 테스트 클래스를 초기화할 때 딱 한번 수행되는 메서드다.
메서드 시그니쳐는 static 으로 선언해야한다.
@BeforeAll
static void beforeAll() {
System.out.println("@BeforeAll");
}
@BeforeEach
본 어노테이션을 붙인 메서드는 테스트 메서드 실행 이전에 수행된다.
@BeforeEach
void beforeEach() {
System.out.println("@BeforeEach");
}
@AfterAll
본 어노테이션을 붙인 메서드는 해당 테스트 클래스 내 테스트 메서드를 모두 실행시킨 후 딱 한번 수행되는 메서드다.
메서드 시그니쳐는 static 으로 선언해야한다.
@AfterAll
static void afterAll() {
System.out.println("@AfterAll");
}
@AfterEach
본 어노테이션을 붙인 메서드는 테스트 메서드 실행 이후에 수행된다.
@AfterEach
void afterEach() {
System.out.println("@AfterEach");
}
@Disabled
본 어노테이션을 붙인 테스트 메서드는 무시된다.
@Disabled
@Test
void create3() {
System.out.println("create3()");
}
코드
/**
* @author : Eunmo Hong
* @since : 2020/08/13
*/
class StudyTest {
@Test
void create1() {
Study study = new Study();
assertNotNull(study);
System.out.println("create1()");
}
@Test
void create2() {
System.out.println("create2()");
}
@Disabled
@Test
void create3() {
System.out.println("create3()");
}
@BeforeAll
static void beforeAll() {
System.out.println("@BeforeAll");
}
@AfterAll
static void afterAll() {
System.out.println("@AfterAll");
}
@BeforeEach
void beforeEach() {
System.out.println("@BeforeEach");
}
@AfterEach
void afterEach() {
System.out.println("@AfterEach");
}
}
실행 콘솔
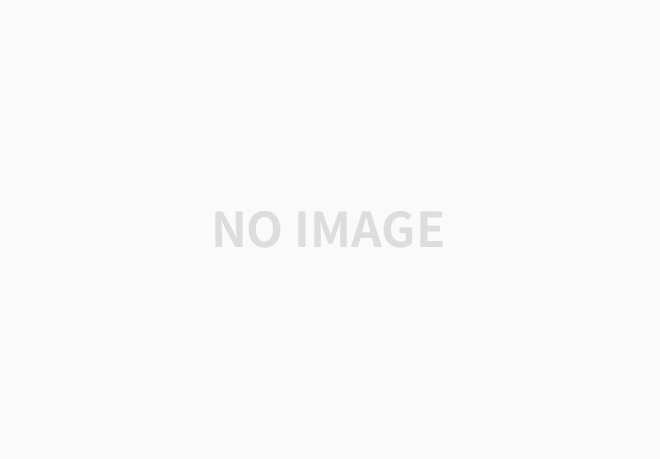
- @BeforeAll 이 실행된다.
--------반복------- @BeforeEach 가 실행된다
- @Test 를 붙인 메서드가 실행된다.
- @AfterEach 가 실행된다
- @AfterAll 이 실행된다
깃헙 주소
반응형
'Test' 카테고리의 다른 글
[JUnit5] assertEquals, assertAll, assertTimeout.... (0) | 2020.08.13 |
---|---|
@SpringBootTest와 @WebMvcTest, @MockBean 그리고 WebClinet까지 (1) | 2020.01.29 |
[JUnit5] 기본 애너테이션들. (0) | 2019.12.11 |